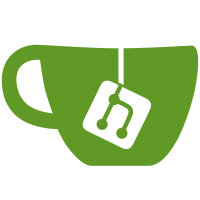
11 changed files with 854 additions and 665 deletions
-
145src/pages/login/register.tsx
-
160src/pages/use/login/index.tsx
-
0src/pages/use/login/style.ts
-
115src/pages/use/password/retrieve.tsx
-
145src/pages/use/register/index.tsx
-
6src/request.ts
-
334src/routes.tsx
-
8src/service/system.ts
-
22src/store/system/user.ts
@ -1,145 +0,0 @@ |
|||||
import { Layout, Input, Button, Typography, Row, Col, Form } from "antd"; |
|
||||
import { LockOutlined, MailOutlined } from "@ant-design/icons"; |
|
||||
import { createFileRoute } from "@tanstack/react-router"; |
|
||||
import { useAtomValue } from "jotai"; |
|
||||
import React, { memo, useEffect, useState } from "react"; |
|
||||
import {emailCodeAtom} from "@/store/system/user.ts"; |
|
||||
const { Title, Text, Link } = Typography; |
|
||||
|
|
||||
const Register = memo(() => { |
|
||||
const [registerForm] = Form.useForm(); |
|
||||
const { mutate: emailCodeMutate } = useAtomValue(emailCodeAtom); |
|
||||
|
|
||||
const handleRegisterSubmit = (values: any) => { |
|
||||
console.log(values); |
|
||||
// 调用注册API
|
|
||||
}; |
|
||||
|
|
||||
const handleGetCode = () => { |
|
||||
const email = registerForm.getFieldValue("email"); |
|
||||
setCountdown(10); |
|
||||
setIsButtonDisabled(true); |
|
||||
emailCodeMutate({ email }); |
|
||||
}; |
|
||||
|
|
||||
const [countdown, setCountdown] = useState<number>(0); |
|
||||
const [isButtonDisabled, setIsButtonDisabled] = useState<boolean>(false); |
|
||||
|
|
||||
useEffect(() => { |
|
||||
let timer: number; |
|
||||
if (countdown > 0) { |
|
||||
timer = setTimeout(() => setCountdown(countdown - 1), 1000); |
|
||||
} else { |
|
||||
setIsButtonDisabled(false); |
|
||||
} |
|
||||
return () => clearTimeout(timer); |
|
||||
}, [countdown]); |
|
||||
|
|
||||
useEffect(() => { |
|
||||
document.body.className = "register"; |
|
||||
return () => { |
|
||||
document.body.className = document.body.className.replace("register", ""); |
|
||||
}; |
|
||||
}, []); |
|
||||
|
|
||||
return ( |
|
||||
<Layout |
|
||||
style={{ |
|
||||
height: "100vh", |
|
||||
background: "url('https://placehold.co/1920x1080') no-repeat center center", |
|
||||
backgroundSize: "cover", |
|
||||
}} |
|
||||
> |
|
||||
<Row justify="center" align="middle" style={{ height: "100%" }}> |
|
||||
<Col> |
|
||||
<Row> |
|
||||
<Col span={12} style={{ padding: "20px" }}> |
|
||||
<Title level={3}>向量检索服务免费试用</Title> |
|
||||
<Text>免费试用向量检索服务,玩转大模型生成式检索</Text> |
|
||||
<br /> |
|
||||
<Link href="#">查看详情 ></Link> |
|
||||
</Col> |
|
||||
<Col |
|
||||
span={12} |
|
||||
style={{ |
|
||||
padding: "20px", |
|
||||
background: "#fff", |
|
||||
borderRadius: "8px", |
|
||||
position: "relative", |
|
||||
width: "500px", |
|
||||
height: "500px", |
|
||||
}} |
|
||||
> |
|
||||
<div |
|
||||
style={{ |
|
||||
position: "absolute", |
|
||||
top: 0, |
|
||||
right: 0, |
|
||||
width: 0, |
|
||||
height: 0, |
|
||||
borderLeft: "80px solid transparent", |
|
||||
borderTop: "80px solid #ff9800", |
|
||||
textAlign: "center", |
|
||||
cursor: "pointer", // 设置手型光标
|
|
||||
}} |
|
||||
> |
|
||||
<text |
|
||||
style={{ |
|
||||
cursor: "pointer", // 设置手型光标
|
|
||||
position: "absolute", |
|
||||
top: "-70px", |
|
||||
right: "0px", |
|
||||
color: "#fff", |
|
||||
fontSize: "16px", |
|
||||
transform: "rotate(0deg)", |
|
||||
textAlign: "center", |
|
||||
width: "60px", |
|
||||
display: "block", |
|
||||
}} |
|
||||
> |
|
||||
注册 |
|
||||
</text> |
|
||||
</div> |
|
||||
<Form form={registerForm} onFinish={handleRegisterSubmit}> |
|
||||
<Form.Item name="email" rules={[{ required: true, message: "请输入邮箱" }]}> |
|
||||
<Input |
|
||||
size="large" |
|
||||
placeholder="请输入邮箱" |
|
||||
prefix={<MailOutlined />} |
|
||||
addonAfter={ |
|
||||
<Button onClick={handleGetCode} disabled={isButtonDisabled}> |
|
||||
{isButtonDisabled ? `${countdown}秒后重试` : "获得验证码"} |
|
||||
</Button> |
|
||||
} |
|
||||
/> |
|
||||
</Form.Item> |
|
||||
<Form.Item name="code" rules={[{ required: true, message: "请输入验证码" }]}> |
|
||||
<Input size="large" placeholder="请输入验证码" prefix={<LockOutlined />} /> |
|
||||
</Form.Item> |
|
||||
<Form.Item name="password" rules={[{ required: true, message: "请输入密码" }]}> |
|
||||
<Input.Password size="large" placeholder="请输入密码" prefix={<LockOutlined />} /> |
|
||||
</Form.Item> |
|
||||
<Form.Item name="confirmPassword" rules={[{ required: true, message: "请确认密码" }]}> |
|
||||
<Input.Password size="large" placeholder="请确认密码" prefix={<LockOutlined />} /> |
|
||||
</Form.Item> |
|
||||
<Button type="primary" htmlType="submit" style={{ width: "100%" }}> |
|
||||
注册 |
|
||||
</Button> |
|
||||
</Form> |
|
||||
<Row justify="space-between" style={{ marginTop: "10px" }}> |
|
||||
<Link href="#">已有账号?登录</Link> |
|
||||
<Link href="#">忘记密码</Link> |
|
||||
</Row> |
|
||||
</Col> |
|
||||
</Row> |
|
||||
</Col> |
|
||||
</Row> |
|
||||
</Layout> |
|
||||
); |
|
||||
}); |
|
||||
|
|
||||
export const Route = createFileRoute("/register")({ |
|
||||
component: Register, |
|
||||
}); |
|
||||
|
|
||||
export default Register; |
|
@ -0,0 +1,115 @@ |
|||||
|
import { Layout, Input, Button, Typography, Row, Col, Form } from "antd"; |
||||
|
import { LockOutlined, MailOutlined, SecurityScanOutlined } from "@ant-design/icons"; |
||||
|
import { createFileRoute, useNavigate } from "@tanstack/react-router"; |
||||
|
import { useAtomValue } from "jotai"; |
||||
|
import React, { memo, useEffect, useState } from "react"; |
||||
|
import { emailCodeAtom } from "@/store/system/user.ts"; |
||||
|
const { Title, Text, Link } = Typography; |
||||
|
|
||||
|
const PwdRetrieve = memo(() => { |
||||
|
const navigate = useNavigate(); |
||||
|
const [retrieveForm] = Form.useForm(); |
||||
|
const { mutate: emailCodeMutate } = useAtomValue(emailCodeAtom); |
||||
|
|
||||
|
const handleRetrieveSubmit = (values: any) => { |
||||
|
console.log(values); |
||||
|
// 调用找回密码API
|
||||
|
}; |
||||
|
|
||||
|
const handleGetCode = () => { |
||||
|
const email = retrieveForm.getFieldValue("email"); |
||||
|
setCountdown(10); |
||||
|
setIsButtonDisabled(true); |
||||
|
emailCodeMutate({ email }); |
||||
|
}; |
||||
|
|
||||
|
const [countdown, setCountdown] = useState<number>(0); |
||||
|
const [isButtonDisabled, setIsButtonDisabled] = useState<boolean>(false); |
||||
|
|
||||
|
useEffect(() => { |
||||
|
let timer: number; |
||||
|
if (countdown > 0) { |
||||
|
timer = setTimeout(() => setCountdown(countdown - 1), 1000); |
||||
|
} else { |
||||
|
setIsButtonDisabled(false); |
||||
|
} |
||||
|
return () => clearTimeout(timer); |
||||
|
}, [countdown]); |
||||
|
|
||||
|
useEffect(() => { |
||||
|
document.body.className = "retrieve"; |
||||
|
return () => { |
||||
|
document.body.className = document.body.className.replace("retrieve", ""); |
||||
|
}; |
||||
|
}, []); |
||||
|
|
||||
|
return ( |
||||
|
<Layout |
||||
|
style={{ |
||||
|
height: "100vh", |
||||
|
background: "url('https://placehold.co/1920x1080') no-repeat center center", |
||||
|
backgroundSize: "cover", |
||||
|
}} |
||||
|
> |
||||
|
<Row justify="center" align="middle" style={{ height: "100%" }}> |
||||
|
<Col> |
||||
|
<Row> |
||||
|
<Col span={12} style={{ padding: "20px" }}> |
||||
|
<Title level={3}>向量检索服务免费试用</Title> |
||||
|
<Text>免费试用向量检索服务,玩转大模型生成式检索</Text> |
||||
|
<br /> |
||||
|
<Link href="#">查看详情 ></Link> |
||||
|
</Col> |
||||
|
<Col |
||||
|
span={12} |
||||
|
style={{ |
||||
|
padding: "20px", |
||||
|
background: "#fff", |
||||
|
borderRadius: "8px", |
||||
|
position: "relative", |
||||
|
width: "500px", |
||||
|
height: "500px", |
||||
|
}} |
||||
|
> |
||||
|
<Form form={retrieveForm} onFinish={handleRetrieveSubmit} style={{ marginTop: "50px" }}> |
||||
|
<Form.Item name="email" rules={[{ required: true, message: "请输入邮箱" }]}> |
||||
|
<Input |
||||
|
size="large" |
||||
|
placeholder="请输入邮箱" |
||||
|
prefix={<MailOutlined />} |
||||
|
addonAfter={ |
||||
|
<Button onClick={handleGetCode} disabled={isButtonDisabled}> |
||||
|
{isButtonDisabled ? `${countdown}秒后重试` : "获得验证码"} |
||||
|
</Button> |
||||
|
} |
||||
|
/> |
||||
|
</Form.Item> |
||||
|
<Form.Item name="code" rules={[{ required: true, message: "请输入验证码" }]}> |
||||
|
<Input size="large" placeholder="请输入验证码" prefix={<SecurityScanOutlined />} /> |
||||
|
</Form.Item> |
||||
|
<Form.Item name="password" rules={[{ required: true, message: "请输入新密码" }]}> |
||||
|
<Input.Password size="large" placeholder="请输入新密码" prefix={<LockOutlined />} /> |
||||
|
</Form.Item> |
||||
|
<Form.Item name="confirmPassword" rules={[{ required: true, message: "请确认新密码" }]}> |
||||
|
<Input.Password size="large" placeholder="请确认新密码" prefix={<LockOutlined />} /> |
||||
|
</Form.Item> |
||||
|
<Button type="primary" htmlType="submit" style={{ width: "100%" }}> |
||||
|
找回密码 |
||||
|
</Button> |
||||
|
</Form> |
||||
|
<Row justify="space-between" style={{ marginTop: "10px" }}> |
||||
|
<Link onClick={() => navigate({ to: "/login" })}>返回登录</Link> |
||||
|
</Row> |
||||
|
</Col> |
||||
|
</Row> |
||||
|
</Col> |
||||
|
</Row> |
||||
|
</Layout> |
||||
|
); |
||||
|
}); |
||||
|
|
||||
|
export const Route = createFileRoute("/pwdRetrieve")({ |
||||
|
component: PwdRetrieve, |
||||
|
}); |
||||
|
|
||||
|
export default PwdRetrieve; |
@ -0,0 +1,145 @@ |
|||||
|
import { Layout, Input, Button, Typography, Row, Col, Form } from "antd"; |
||||
|
import { LockOutlined, MailOutlined, SecurityScanOutlined } from "@ant-design/icons"; |
||||
|
import { createFileRoute, useNavigate } from "@tanstack/react-router"; |
||||
|
import { useAtomValue } from "jotai"; |
||||
|
import React, { memo, useEffect, useState } from "react"; |
||||
|
import { emailCodeAtom } from "@/store/system/user.ts"; |
||||
|
const { Title, Text, Link } = Typography; |
||||
|
|
||||
|
const Register = memo(() => { |
||||
|
const navigate = useNavigate(); |
||||
|
const [registerForm] = Form.useForm(); |
||||
|
const { mutate: emailCodeMutate } = useAtomValue(emailCodeAtom); |
||||
|
|
||||
|
const handleRegisterSubmit = (values: any) => { |
||||
|
console.log(values); |
||||
|
// 调用注册API
|
||||
|
}; |
||||
|
|
||||
|
const handleGetCode = () => { |
||||
|
const email = registerForm.getFieldValue("email"); |
||||
|
setCountdown(10); |
||||
|
setIsButtonDisabled(true); |
||||
|
emailCodeMutate({ email }); |
||||
|
}; |
||||
|
|
||||
|
const [countdown, setCountdown] = useState<number>(0); |
||||
|
const [isButtonDisabled, setIsButtonDisabled] = useState<boolean>(false); |
||||
|
|
||||
|
useEffect(() => { |
||||
|
let timer: number; |
||||
|
if (countdown > 0) { |
||||
|
timer = setTimeout(() => setCountdown(countdown - 1), 1000); |
||||
|
} else { |
||||
|
setIsButtonDisabled(false); |
||||
|
} |
||||
|
return () => clearTimeout(timer); |
||||
|
}, [countdown]); |
||||
|
|
||||
|
useEffect(() => { |
||||
|
document.body.className = "register"; |
||||
|
return () => { |
||||
|
document.body.className = document.body.className.replace("register", ""); |
||||
|
}; |
||||
|
}, []); |
||||
|
|
||||
|
return ( |
||||
|
<Layout |
||||
|
style={{ |
||||
|
height: "100vh", |
||||
|
background: "url('https://placehold.co/1920x1080') no-repeat center center", |
||||
|
backgroundSize: "cover", |
||||
|
}} |
||||
|
> |
||||
|
<Row justify="center" align="middle" style={{ height: "100%" }}> |
||||
|
<Col> |
||||
|
<Row> |
||||
|
<Col span={12} style={{ padding: "20px" }}> |
||||
|
<Title level={3}>向量检索服务免费试用</Title> |
||||
|
<Text>免费试用向量检索服务,玩转大模型生成式检索</Text> |
||||
|
<br /> |
||||
|
<Link href="#">查看详情 ></Link> |
||||
|
</Col> |
||||
|
<Col |
||||
|
span={12} |
||||
|
style={{ |
||||
|
padding: "20px", |
||||
|
background: "#fff", |
||||
|
borderRadius: "8px", |
||||
|
position: "relative", |
||||
|
width: "500px", |
||||
|
height: "500px", |
||||
|
}} |
||||
|
> |
||||
|
<Form form={registerForm} onFinish={handleRegisterSubmit} style={{ marginTop: "50px" }}> |
||||
|
<Form.Item name="email" rules={[{ required: true, message: "请输入邮箱" }]}> |
||||
|
<Input |
||||
|
size="large" |
||||
|
placeholder="请输入邮箱" |
||||
|
prefix={<MailOutlined />} |
||||
|
addonAfter={ |
||||
|
<Button onClick={handleGetCode} disabled={isButtonDisabled}> |
||||
|
{isButtonDisabled ? `${countdown}秒后重试` : "获得验证码"} |
||||
|
</Button> |
||||
|
} |
||||
|
/> |
||||
|
</Form.Item> |
||||
|
<Form.Item name="code" rules={[{ required: true, message: "请输入验证码" }]}> |
||||
|
<Input size="large" placeholder="请输入验证码" prefix={<SecurityScanOutlined />} /> |
||||
|
</Form.Item> |
||||
|
<Form.Item name="password" rules={[{ required: true, message: "请输入密码" }]}> |
||||
|
<Input.Password size="large" placeholder="请输入密码" prefix={<LockOutlined />} /> |
||||
|
</Form.Item> |
||||
|
<Form.Item name="confirmPassword" rules={[{ required: true, message: "请确认密码" }]}> |
||||
|
<Input.Password size="large" placeholder="请确认密码" prefix={<LockOutlined />} /> |
||||
|
</Form.Item> |
||||
|
<Button type="primary" htmlType="submit" style={{ width: "100%" }}> |
||||
|
注册 |
||||
|
</Button> |
||||
|
</Form> |
||||
|
<div |
||||
|
style={{ |
||||
|
position: "absolute", |
||||
|
top: 0, |
||||
|
right: 0, |
||||
|
width: 0, |
||||
|
height: 0, |
||||
|
borderLeft: "80px solid transparent", |
||||
|
borderTop: "80px solid #ff9800", |
||||
|
textAlign: "center", |
||||
|
}} |
||||
|
> |
||||
|
<span |
||||
|
style={{ |
||||
|
cursor: "pointer", // 设置手型光标
|
||||
|
position: "absolute", |
||||
|
top: "-70px", |
||||
|
right: "0px", |
||||
|
color: "#fff", |
||||
|
fontSize: "16px", |
||||
|
transform: "rotate(0deg)", |
||||
|
textAlign: "center", |
||||
|
width: "60px", |
||||
|
display: "block", |
||||
|
}} |
||||
|
onClick={() => navigate({ to: "/login" })} |
||||
|
> |
||||
|
登录 |
||||
|
</span> |
||||
|
</div> |
||||
|
<Row justify="space-between" style={{ marginTop: "10px" }}> |
||||
|
<Link onClick={() => navigate({ to: "/login" })}>返回登录</Link> |
||||
|
</Row> |
||||
|
</Col> |
||||
|
</Row> |
||||
|
</Col> |
||||
|
</Row> |
||||
|
</Layout> |
||||
|
); |
||||
|
}); |
||||
|
|
||||
|
export const Route = createFileRoute("/register")({ |
||||
|
component: Register, |
||||
|
}); |
||||
|
|
||||
|
export default Register; |
Write
Preview
Loading…
Cancel
Save
Reference in new issue