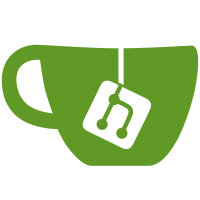
11 changed files with 508 additions and 1 deletions
-
19src/service/cms.ts
-
86src/store/cms/collect.ts
-
87src/store/cms/video.ts
-
87src/store/cms/video_cloud.ts
-
87src/store/cms/video_magnet.ts
-
21src/types/cms/collect.d.ts
-
38src/types/cms/video.d.ts
-
38src/types/cms/video_cloud.d.ts
-
37src/types/cms/video_magnet.d.ts
-
7src/types/index.d.ts
@ -0,0 +1,19 @@ |
|||
import { createCURD } from '@/service/base.ts' |
|||
import { Cms } from '@/types' |
|||
|
|||
const cmsServ = { |
|||
collect: { |
|||
...createCURD<any, Cms.ICollect>('/cms/collect') |
|||
}, |
|||
video: { |
|||
...createCURD<any, Cms.IVideo>('/cms/video') |
|||
}, |
|||
videoCloud: { |
|||
...createCURD<any, Cms.IVideoCloud>('/cms/video_cloud') |
|||
}, |
|||
videoMagnet: { |
|||
...createCURD<any, Cms.IVideoMagnet>('/cms/video_magnet') |
|||
}, |
|||
} |
|||
|
|||
export default cmsServ |
@ -0,0 +1,86 @@ |
|||
import { Cms } from '@/types' |
|||
import { atom } from 'jotai' |
|||
import { IApiResult, IPage } from '@/global' |
|||
import { atomWithMutation, atomWithQuery, queryClientAtom } from 'jotai-tanstack-query' |
|||
import { message } from 'antd' |
|||
import { t } from 'i18next' |
|||
import cmsServ from '@/service/cms.ts' |
|||
|
|||
|
|||
type SearchParams = IPage & { |
|||
key?: string |
|||
} |
|||
|
|||
export const idAtom = atom(0) |
|||
|
|||
export const collectIdsAtom = atom<number[]>([]) |
|||
|
|||
export const collectAtom = atom<Cms.ICollect>(undefined as unknown as Cms.ICollect) |
|||
|
|||
export const searchAtom = atom<SearchParams>({ |
|||
key: '' |
|||
} as SearchParams) |
|||
|
|||
export const pageAtom = atom<IPage>({ |
|||
pageSize: 10, |
|||
page: 1, |
|||
}) |
|||
|
|||
export const collectsAtom = atomWithQuery((get) => { |
|||
return { |
|||
queryKey: [ 'collects', get(searchAtom) ], |
|||
queryFn: async ({ queryKey: [ , params ] }) => { |
|||
return await cmsServ.collect.list(params as SearchParams) |
|||
}, |
|||
select: res => { |
|||
const data = res.data |
|||
data.rows = data.rows?.map(row => { |
|||
return { |
|||
...row, |
|||
} |
|||
}) |
|||
return data |
|||
} |
|||
} |
|||
}) |
|||
|
|||
//saveOrUpdateAtom
|
|||
export const saveOrUpdateCollectAtom = atomWithMutation<IApiResult, Cms.ICollect>((get) => { |
|||
|
|||
return { |
|||
mutationKey: [ 'updateCollect' ], |
|||
mutationFn: async (data) => { |
|||
// data.status = data.status ? '1' : '0'
|
|||
if (data.id === 0) { |
|||
return await cmsServ.collect.add(data) |
|||
} |
|||
return await cmsServ.collect.update(data) |
|||
}, |
|||
onSuccess: (res) => { |
|||
const isAdd = !!res.data?.id |
|||
message.success(t(isAdd ? 'message.saveSuccess' : 'message.editSuccess', '保存成功')) |
|||
|
|||
//更新列表
|
|||
// eslint-disable-next-line @typescript-eslint/ban-ts-comment
|
|||
// @ts-ignore fix
|
|||
get(queryClientAtom).invalidateQueries({ queryKey: [ 'collects', get(searchAtom) ] }) |
|||
|
|||
return res |
|||
} |
|||
} |
|||
}) |
|||
|
|||
export const deleteCollectAtom = atomWithMutation((get) => { |
|||
return { |
|||
mutationKey: [ 'deleteCollect' ], |
|||
mutationFn: async (ids: number[]) => { |
|||
return await cmsServ.collect.batchDelete(ids ?? get(collectIdsAtom)) |
|||
}, |
|||
onSuccess: (res) => { |
|||
message.success('message.deleteSuccess') |
|||
//更新列表
|
|||
get(queryClientAtom).invalidateQueries({ queryKey: [ 'collects', get(searchAtom) ] }) |
|||
return res |
|||
} |
|||
} |
|||
}) |
@ -0,0 +1,87 @@ |
|||
import { atom } from 'jotai' |
|||
import { IApiResult, IPage } from '@/global' |
|||
import { atomWithMutation, atomWithQuery, queryClientAtom } from 'jotai-tanstack-query' |
|||
import { message } from 'antd' |
|||
import { t } from 'i18next' |
|||
import { Cms } from '@/types' |
|||
import cmsServ from '@/service/cms.ts' |
|||
|
|||
|
|||
type SearchParams = IPage & { |
|||
key?: string |
|||
} |
|||
|
|||
export const idAtom = atom(0) |
|||
|
|||
export const videoIdsAtom = atom<number[]>([]) |
|||
|
|||
export const videoAtom = atom<Cms.IVideo>(undefined as unknown as Cms.IVideo) |
|||
|
|||
export const searchAtom = atom<SearchParams>({ |
|||
key: '' |
|||
} as SearchParams) |
|||
|
|||
export const pageAtom = atom<IPage>({ |
|||
pageSize: 10, |
|||
page: 1, |
|||
}) |
|||
|
|||
export const videosAtom = atomWithQuery((get) => { |
|||
return { |
|||
queryKey: [ 'videos', get(searchAtom) ], |
|||
queryFn: async ({ queryKey: [ , params ] }) => { |
|||
return await cmsServ.video.list(params as SearchParams) |
|||
}, |
|||
select: res => { |
|||
const data = res.data |
|||
data.rows = data.rows?.map(row => { |
|||
return { |
|||
...row, |
|||
//status: convertToBool(row.status)
|
|||
} |
|||
}) |
|||
return data |
|||
} |
|||
} |
|||
}) |
|||
|
|||
//saveOrUpdateAtom
|
|||
export const saveOrUpdateVideoAtom = atomWithMutation<IApiResult, Cms.IVideo>((get) => { |
|||
|
|||
return { |
|||
mutationKey: [ 'updateVideo' ], |
|||
mutationFn: async (data) => { |
|||
//data.status = data.status ? '1' : '0'
|
|||
if (data.id === 0) { |
|||
return await cmsServ.video.add(data) |
|||
} |
|||
return await cmsServ.video.update(data) |
|||
}, |
|||
onSuccess: (res) => { |
|||
const isAdd = !!res.data?.id |
|||
message.success(t(isAdd ? 'message.saveSuccess' : 'message.editSuccess', '保存成功')) |
|||
|
|||
//更新列表
|
|||
// eslint-disable-next-line @typescript-eslint/ban-ts-comment
|
|||
// @ts-ignore fix
|
|||
get(queryClientAtom).invalidateQueries({ queryKey: [ 'videos', get(searchAtom) ] }) |
|||
|
|||
return res |
|||
} |
|||
} |
|||
}) |
|||
|
|||
export const deleteVideoAtom = atomWithMutation((get) => { |
|||
return { |
|||
mutationKey: [ 'deleteVideo' ], |
|||
mutationFn: async (ids: number[]) => { |
|||
return await cmsServ.video.batchDelete(ids ?? get(videoIdsAtom)) |
|||
}, |
|||
onSuccess: (res) => { |
|||
message.success('message.deleteSuccess') |
|||
//更新列表
|
|||
get(queryClientAtom).invalidateQueries({ queryKey: [ 'videos', get(searchAtom) ] }) |
|||
return res |
|||
} |
|||
} |
|||
}) |
@ -0,0 +1,87 @@ |
|||
import { atom } from 'jotai' |
|||
import { IApiResult, IPage } from '@/global' |
|||
import { atomWithMutation, atomWithQuery, queryClientAtom } from 'jotai-tanstack-query' |
|||
import { message } from 'antd' |
|||
import { t } from 'i18next' |
|||
import { Cms } from '@/types' |
|||
import cmsServ from '@/service/cms.ts' |
|||
|
|||
|
|||
type SearchParams = IPage & { |
|||
key?: string |
|||
} |
|||
|
|||
export const idAtom = atom(0) |
|||
|
|||
export const videoCloudIdsAtom = atom<number[]>([]) |
|||
|
|||
export const videoCloudAtom = atom<Cms.IVideoCloud>(undefined as unknown as Cms.IVideoCloud) |
|||
|
|||
export const searchAtom = atom<SearchParams>({ |
|||
key: '' |
|||
} as SearchParams) |
|||
|
|||
export const pageAtom = atom<IPage>({ |
|||
pageSize: 10, |
|||
page: 1, |
|||
}) |
|||
|
|||
export const videoCloudsAtom = atomWithQuery((get) => { |
|||
return { |
|||
queryKey: [ 'videoClouds', get(searchAtom) ], |
|||
queryFn: async ({ queryKey: [ , params ] }) => { |
|||
return await cmsServ.videoCloud.list(params as SearchParams) |
|||
}, |
|||
select: res => { |
|||
const data = res.data |
|||
data.rows = data.rows?.map(row => { |
|||
return { |
|||
...row, |
|||
//status: convertToBool(row.status)
|
|||
} |
|||
}) |
|||
return data |
|||
} |
|||
} |
|||
}) |
|||
|
|||
//saveOrUpdateAtom
|
|||
export const saveOrUpdateVideoCloudAtom = atomWithMutation<IApiResult, Cms.IVideoCloud>((get) => { |
|||
|
|||
return { |
|||
mutationKey: [ 'updateVideoCloud' ], |
|||
mutationFn: async (data) => { |
|||
//data.status = data.status ? '1' : '0'
|
|||
if (data.id === 0) { |
|||
return await cmsServ.videoCloud.add(data) |
|||
} |
|||
return await cmsServ.videoCloud.update(data) |
|||
}, |
|||
onSuccess: (res) => { |
|||
const isAdd = !!res.data?.id |
|||
message.success(t(isAdd ? 'message.saveSuccess' : 'message.editSuccess', '保存成功')) |
|||
|
|||
//更新列表
|
|||
// eslint-disable-next-line @typescript-eslint/ban-ts-comment
|
|||
// @ts-ignore fix
|
|||
get(queryClientAtom).invalidateQueries({ queryKey: [ 'videoClouds', get(searchAtom) ] }) |
|||
|
|||
return res |
|||
} |
|||
} |
|||
}) |
|||
|
|||
export const deleteVideoCloudAtom = atomWithMutation((get) => { |
|||
return { |
|||
mutationKey: [ 'deleteVideoCloud' ], |
|||
mutationFn: async (ids: number[]) => { |
|||
return await cmsServ.videoCloud.batchDelete(ids ?? get(videoCloudIdsAtom)) |
|||
}, |
|||
onSuccess: (res) => { |
|||
message.success('message.deleteSuccess') |
|||
//更新列表
|
|||
get(queryClientAtom).invalidateQueries({ queryKey: [ 'videoClouds', get(searchAtom) ] }) |
|||
return res |
|||
} |
|||
} |
|||
}) |
@ -0,0 +1,87 @@ |
|||
import { atom } from 'jotai' |
|||
import { IApiResult, IPage } from '@/global' |
|||
import { atomWithMutation, atomWithQuery, queryClientAtom } from 'jotai-tanstack-query' |
|||
import { message } from 'antd' |
|||
import { t } from 'i18next' |
|||
import { Cms } from '@/types' |
|||
import cmsServ from '@/service/cms.ts' |
|||
|
|||
|
|||
type SearchParams = IPage & { |
|||
key?: string |
|||
} |
|||
|
|||
export const idAtom = atom(0) |
|||
|
|||
export const videoMagnetIdsAtom = atom<number[]>([]) |
|||
|
|||
export const videoMagnetAtom = atom<Cms.IVideoMagnet>(undefined as unknown as Cms.IVideoMagnet) |
|||
|
|||
export const searchAtom = atom<SearchParams>({ |
|||
key: '' |
|||
} as SearchParams) |
|||
|
|||
export const pageAtom = atom<IPage>({ |
|||
pageSize: 10, |
|||
page: 1, |
|||
}) |
|||
|
|||
export const videoMagnetsAtom = atomWithQuery((get) => { |
|||
return { |
|||
queryKey: [ 'videoMagnets', get(searchAtom) ], |
|||
queryFn: async ({ queryKey: [ , params ] }) => { |
|||
return await cmsServ.videoMagnet.list(params as SearchParams) |
|||
}, |
|||
select: res => { |
|||
const data = res.data |
|||
data.rows = data.rows?.map(row => { |
|||
return { |
|||
...row, |
|||
//status: convertToBool(row.status)
|
|||
} |
|||
}) |
|||
return data |
|||
} |
|||
} |
|||
}) |
|||
|
|||
//saveOrUpdateAtom
|
|||
export const saveOrUpdateVideoMagnetAtom = atomWithMutation<IApiResult, Cms.IVideoMagnet>((get) => { |
|||
|
|||
return { |
|||
mutationKey: [ 'updateVideoMagnet' ], |
|||
mutationFn: async (data) => { |
|||
//data.status = data.status ? '1' : '0'
|
|||
if (data.id === 0) { |
|||
return await cmsServ.videoMagnet.add(data) |
|||
} |
|||
return await cmsServ.videoMagnet.update(data) |
|||
}, |
|||
onSuccess: (res) => { |
|||
const isAdd = !!res.data?.id |
|||
message.success(t(isAdd ? 'message.saveSuccess' : 'message.editSuccess', '保存成功')) |
|||
|
|||
//更新列表
|
|||
// eslint-disable-next-line @typescript-eslint/ban-ts-comment
|
|||
// @ts-ignore fix
|
|||
get(queryClientAtom).invalidateQueries({ queryKey: [ 'videoMagnets', get(searchAtom) ] }) |
|||
|
|||
return res |
|||
} |
|||
} |
|||
}) |
|||
|
|||
export const deleteVideoMagnetAtom = atomWithMutation((get) => { |
|||
return { |
|||
mutationKey: [ 'deleteVideoMagnet' ], |
|||
mutationFn: async (ids: number[]) => { |
|||
return await cmsServ.videoMagnet.batchDelete(ids ?? get(videoMagnetIdsAtom)) |
|||
}, |
|||
onSuccess: (res) => { |
|||
message.success('message.deleteSuccess') |
|||
//更新列表
|
|||
get(queryClientAtom).invalidateQueries({ queryKey: [ 'videoMagnets', get(searchAtom) ] }) |
|||
return res |
|||
} |
|||
} |
|||
}) |
@ -0,0 +1,21 @@ |
|||
export interface ICollect { |
|||
id: number; |
|||
name: string; |
|||
url: string; |
|||
param: string; |
|||
type_id: number; |
|||
opt: number; |
|||
filter: number; |
|||
filter_form: string; |
|||
sync_pic: number; |
|||
icon_cdn: string; |
|||
class: string; |
|||
weights: number; |
|||
status: boolean; |
|||
open_replace: boolean; |
|||
replace_str: string; |
|||
site_auth: boolean; |
|||
site_cooperation: boolean; |
|||
categories_rules: string; |
|||
|
|||
} |
@ -0,0 +1,38 @@ |
|||
export interface IVideo { |
|||
id: number; |
|||
source_url: string; |
|||
collect_id: number; |
|||
type_id: number; |
|||
title: string; |
|||
title_sub: string; |
|||
letter: string; |
|||
tag: string; |
|||
lock: number; |
|||
copyright: number; |
|||
is_end: number; |
|||
status: number; |
|||
category_id: number; |
|||
pic: string; |
|||
pic_local: string; |
|||
pic_status: number; |
|||
actor: string; |
|||
director: string; |
|||
writer: string; |
|||
remarks: string; |
|||
pubdate: string; |
|||
total: number; |
|||
serial: string; |
|||
duration: string; |
|||
area: string; |
|||
lang: string; |
|||
version: string; |
|||
year: number; |
|||
state: string; |
|||
douban_score: number; |
|||
douban_id: number; |
|||
imdb_score: number; |
|||
imdb_id: number; |
|||
content: string; |
|||
created_at: any; |
|||
updated_at: any; |
|||
} |
@ -0,0 +1,38 @@ |
|||
|
|||
export interface IVideoCloud { |
|||
id: number; |
|||
type_id: number; |
|||
collect_id: number; |
|||
source_url: string; |
|||
title: string; |
|||
title_sub: string; |
|||
letter: string; |
|||
tag: string; |
|||
lock: number; |
|||
copyright: number; |
|||
is_end: number; |
|||
status: number; |
|||
category_id: number; |
|||
pic: string; |
|||
pic_local: string; |
|||
actor: string; |
|||
director: string; |
|||
writer: string; |
|||
content: string; |
|||
pubdate: string; |
|||
total: number; |
|||
serial: string; |
|||
duration: string; |
|||
class: string; |
|||
area: string; |
|||
lang: string; |
|||
version: string; |
|||
year: number; |
|||
state: string; |
|||
douban_score: number; |
|||
douban_id: number; |
|||
imdb_score: number; |
|||
imdb_id: number; |
|||
created_at: any; |
|||
updated_at: any; |
|||
} |
@ -0,0 +1,37 @@ |
|||
export interface IVideoMagnet { |
|||
id: number; |
|||
type_id: number; |
|||
collect_id: number; |
|||
source_url: string; |
|||
title: string; |
|||
title_sub: string; |
|||
letter: string; |
|||
tag: string; |
|||
lock: number; |
|||
copyright: number; |
|||
is_end: number; |
|||
status: number; |
|||
category_id: number; |
|||
pic: string; |
|||
pic_local: string; |
|||
actor: string; |
|||
director: string; |
|||
writer: string; |
|||
content: string; |
|||
pubdate: string; |
|||
total: number; |
|||
serial: string; |
|||
duration: string; |
|||
class: string; |
|||
area: string; |
|||
lang: string; |
|||
version: string; |
|||
year: number; |
|||
state: string; |
|||
douban_score: number; |
|||
douban_id: number; |
|||
imdb_score: number; |
|||
imdb_id: number; |
|||
created_at: any; |
|||
updated_at: any; |
|||
} |
Write
Preview
Loading…
Cancel
Save
Reference in new issue